Upload form data with file using Multer & MYSQL in NextJs
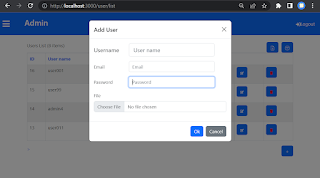
The previous tutorial taught you how to create API endpoints to filter MYSQL database, pagination, and protect the API using middleware. This tutorial teaches you how to upload user form data with image file using Multer and delete data from MYSQL database. From the project folder, execute the following command to install Multer: npm install multer Then open api/user/[slug].js file to add post, put, and delete routes. To use multer to parse request body and upload files, you need to disable default body parser by setting bodyParser to false. We use diskStorage() function from multer to configure local storage and file name. Multer needs file field and max count of the file to be uploaded. In this project, we use array() function to specify file field (photo) and the max count of the file to be uploaded (1). We can access text parts of a submitted form data by extracting body from the request. To access file parts, you need to extract files from the request. ..... import bcrypt